To get the report for of the users using native method
Open the site you want to report on.
Click the cog icon to open the Settings menu and then click Site usage.
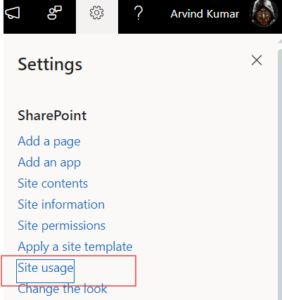
Scroll down to the Shared with external users section and click Run report.
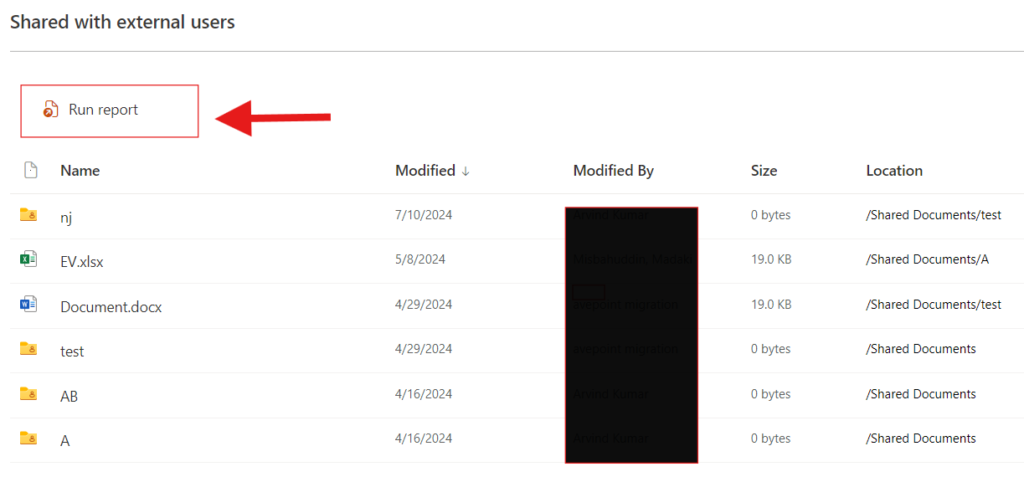
Pick a location to save the report and click Run report.
Browse to the SharePoint Online location where you chose to save the report.
Review the CSV report in Excel, filtering out default records (such as site Owners, Members and Visitors)

The above method will provide the details based on Site collection basis, if you want to extract the details of the internal and external user for all the sites in the tenant you can use the PowerShell command mentioned below
Prerequisites
- PowerShell Environment:
- Ensure you have PowerShell installed on your machine. For best results, use PowerShell 5.1 or later.
- PnP PowerShell Module:
- This script uses the PnP PowerShell module, which simplifies working with SharePoint Online. Install it using the command:
Install-Module -Name PnP.PowerShell -Scope CurrentUser
- This script uses the PnP PowerShell module, which simplifies working with SharePoint Online. Install it using the command:
- Permissions:
- Ensure you have administrative rights to access the SharePoint Online Admin Center and the site collections within it.
# Config Variables
$AdminURL = "https://contoso-admin.sharepoint.com" # Replace with your SharePoint Online Admin URL
$CSVFile = "C:\Temp\arvindtest.csv"
# Function to Connect to SharePoint Online
Function Connect-ToSite {
param (
[string]$SiteURL,
[System.Management.Automation.PSCredential]$Credential
)
# Connect to PnP Online
Connect-PnPOnline -Url $SiteURL -Credentials $Credential -ErrorAction Stop
}
# Prompt for Credentials
$Credential = Get-Credential
# Connect to the SharePoint Online Admin Center
Connect-PnPOnline -Url $AdminURL -Credentials $Credential -ErrorAction Stop
# Get all site collections
$SiteCollections = Get-PnPTenantSite -ErrorAction Stop
# Arrays to store different types of users
$InternalUsers = @()
$ExternalUsers = @()
$GuestUsers = @()
# Loop through each site collection
foreach ($Site in $SiteCollections) {
# Exclude OneDrive sites by checking if the URL contains "my.sharepoint.com"
if ($Site.Url -notlike "*my.sharepoint.com*") {
Write-Host "Processing site collection: $($Site.Url)"
# Connect to the site collection
Connect-ToSite -SiteURL $Site.Url -Credential $Credential
# Get all users of the site collection
$Users = Get-PnPUser
# Loop through Users and categorize them
foreach ($User in $Users) {
# Determine user type
$UserType = switch -Wildcard ($User.LoginName) {
"*#ext#*" { "External" }
"*#guest#*" { "Guest" }
default { "Internal" }
}
# Create user object
$UserObject = [PSCustomObject]@{
"Site URL" = $Site.Url
"User Name" = $User.Title
"Login ID" = $User.LoginName
"E-mail" = $User.Email
"User Type" = $UserType
}
# Add to appropriate array
switch ($UserType) {
"Internal" { $InternalUsers += $UserObject }
"External" { $ExternalUsers += $UserObject }
"Guest" { $GuestUsers += $UserObject }
}
}
} else {
Write-Host "Skipping OneDrive site: $($Site.Url)"
}
}
# Export Users data to CSV file
$AllUsers = $InternalUsers + $ExternalUsers + $GuestUsers
$AllUsers | Export-Csv -NoTypeInformation -Path $CSVFile
Write-Host "User data exported to $CSVFile"
Output
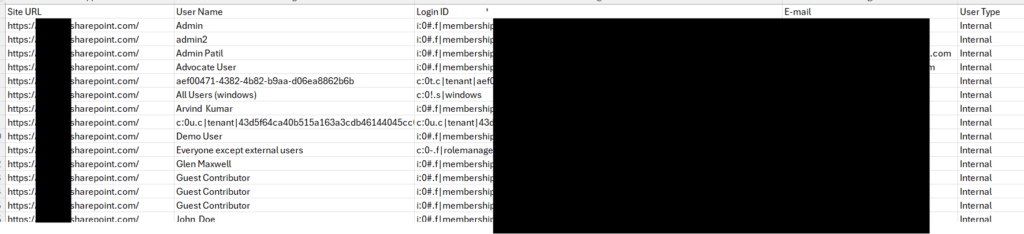